Password Protection Pt. 2: Backspacing (and String Functions)
In the previous Password Protection Tutorial, we explored a simple solution to creating a password entry prompt that enables a certain degree of privacy when accessing their flash lite menu. I have received a couple responses from users wanting to know how to create a backspace function so that if the user accidentally keys in the wrong digit, they can 'backspace' and re enter something new. Here is what I'm talking about:
Click the swf and press 15403 and press enter. It will display an incorrect password message.
Now type in 15403, then press the left key, then press numpad digit 2. It will remove the last digit you entered and append on the correct one instead. This backspace function can be easily created by considering a couple String functions that come with flash lite 1.1
There are 2 functions we will use: length() and substring().
The length(string) function returns an integer representing the length of the string you have given it. For instance:
theWord="apple"
numLetters = length(theWord);
trace(numLetters); //OUTPUT will be 5
The substring(string,index,count) function returns a string variable.
string
The string from which to extract the new string.index
The number of the first character to extract.count
The number of characters to include in the extracted string, not including the index character.
theWord1=substring(theWord,0,3);
trace(theWord1); //OUTPUT will be 'sna'
The Backspace Function
The backspace function is a great example of how these two string functions can be used together. Pressing the backspace button once, will essentially run a substring function that returns a string that is one character shorter. The one liner for this is essentially:
//we only want this to occur when the variable has characters in it
if(length(/:realPW)>0){
/:realPW = substring(/:realPW, 0, length(/:realPW)-1);
}
Implementation into Password Protection
Continuing from our previous password protection tutorial, select the button you had placed on stage that listens to the buttons pressed. Right click and click Actions... At the very bottom at the following code:
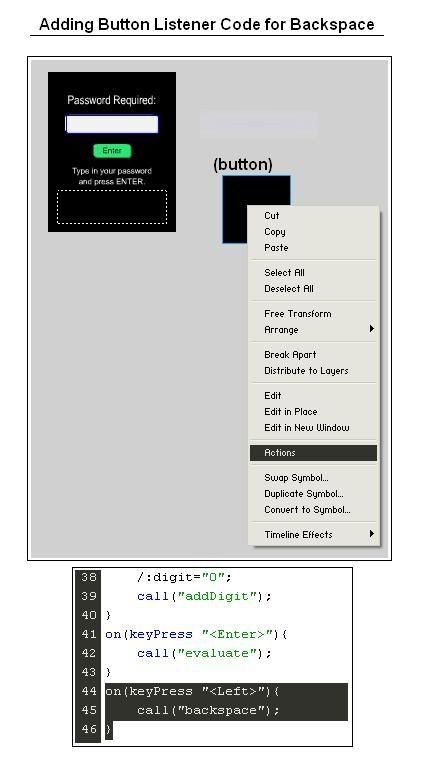
//we designate the 'backspace' button to the left key, but you can change it to whatever you want
on(keyPress "
call("backspace");
}
'backspace' corresponds to a pseudo function we will create. In the area of the timeline designated to these pseudo functions, create a new blank keyframe and give it a frame label of 'backspace'. Right click the blank keyframe and choose Actions... Enter the following:
if(length(/:realPW)>0){
/:realPW = substring(/:realPW, 0, length(/:realPW)-1);
}
if(length(/:pwBox)>0){
/:pwBox = substring(/:pwBox, 0, length(/:pwBox)-1);
}
gotoAndPlay(1);
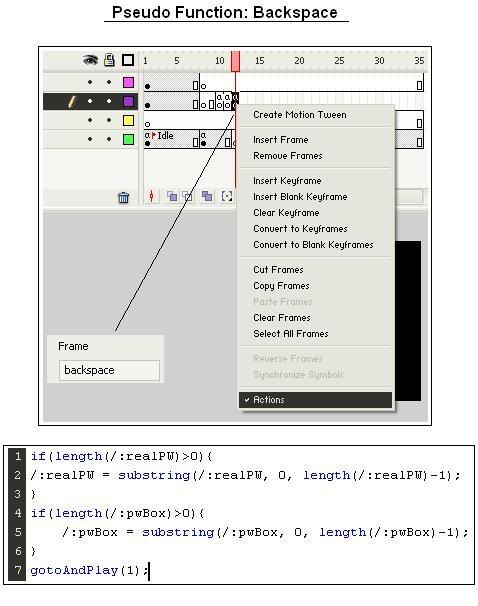
We need to execute the code for both the entered password and the corresponding asterisks that appear on screen. If we don't do this, the number of asterisks on screen would not match up with the number of keystrokes entered.
There you have it! Now you can backspace passwords you enter.
1 comment:
Hi,
Could you please port some flash lite wallpapers to W300 resolution? They work fine in their original ones, but don't look as good as it would if they were 128x160. (same issue on menus @ 176x220)
Post a Comment